What is a CustomComponent
CustomComponent is a type of React Component, which is what goes in every slot of the Ollie Shop Checkout.
A CustomComponent can receive the following props:
cart
: A JSON with information about your cartstoreId
: Id of your store in Ollie ShopshowAlert
: It's a function that you can use to show an alertaddItemsAction
: It's a server function to add an item to the cartupdateItemsAction
: It's a server function for updating an item on the cartupdateCustomerAction
: It's a server function for updating the information of the customerupdatePaymentAction
: it's a server function for updating the Payment dataupdateShippingAction
: It's a server function for updating the Shipping dataupdateDeliveryAction
: It's a server function for updating the Delivery dataupdateExtensionAction
: It's a server function to include extra information to your cart
export const ItemsWithShipping: CustomComponent = ({ cart, updateItemsAction, storeId}) => {
Show Alert
title?: string;
message?: string;
type: "success" | "error" | "warning" | "info";
timeout?: number;
title
: The title that will show for the client
message
: The message that will show for the client
type
: The styles that will be used for this alert
timeout
: For how long this warning will be displayed
export const ExampleComponent: CustomComponent = ({ cart, showAlert}) => {
function showWarning(){
showAlert({
title: "Example Title",
message: "It is an example of description",
type: "info",
timeout: 2000,
})
}
Add Items Action
id: string;
quantity: number;
attributes?: AddItemAttributeDto[];
id
: The id of the SKU
quantity
: the quantity of the SKU to be added to the cart
attributes
is an optional prop that can include metadata of the item, such as Seller information
export const ExampleComponent: CustomComponent = ({ cart, addItemsAction}) => {
function addItemToCart(){
addItemsAction(cart.id, [{
id: "1",
quantity: 1,
attributes: [{
key: "sellerId",
value: "sellerX"
}]
}])
}
Update Items Action
lineId: string;
quantity: number;
lineId
: is a unique ID specific of the Ollie API that refers to the specific item in your cart (serving similar purpose as an index).
quantity
: the quantity of the SKU to be added to the cart
attributes
is an optional prop that can include metadata of the item, such as Seller information
export const ExampleComponent: CustomComponent = ({ cart, updateItemsAction}) => {
function updateItems(){
updateItemsAction(cart.id, [
{
lineId: cart.items[0].lineId,
quantity: 0
}
])
}
Update Customer Action
id?: string;
firstName?: string;
lastName?: string;
email?: string;
phone?: string;
document?: string;
id
: user IDfirstName
: The first Name of the customerlastName
: The last name of the customeremail
: email of the customerphone
: phone number of the customerdocument
: document of the customer
export const ExampleComponent: CustomComponent = ({ cart, updateCustomerAction}) => {
function updateCustomer(){
updateCustomerAction(cart.id, {
email: "[email protected]",
firstName: "First",
lastName: "Last",
phone: "3648412283",
document: "62019792"
})
}
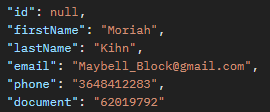
Update Payment Action
selected: [{
id: string;
value: number;
referenceValue: number;
planId?: string;
}]
Id
: Id of the payment method
value
: The amount to be paid with this method (you can send one more object by making a payment with two methods)
referenceValue
: Value of the transaction (for example if you need to include interest or payment fee's)
planId
: Id of the payment plan (for example there might be multiple options to choose from the same payment method)
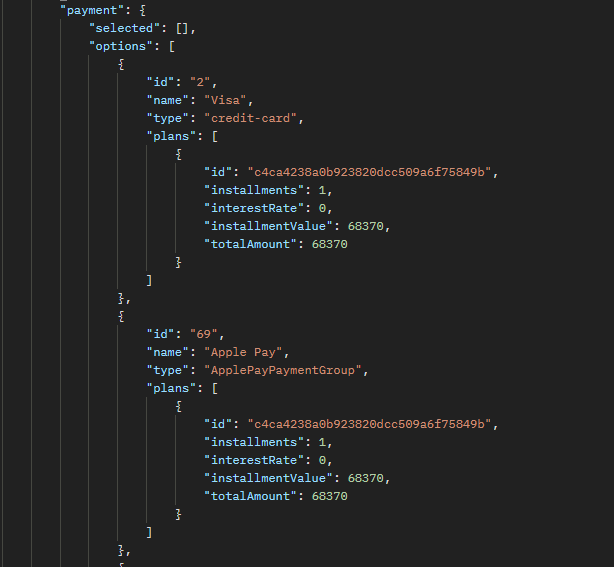
export const ExampleComponent: CustomComponent = ({ cart, updatePaymentAction}) => {
function updateCustomer(){
updatePaymentAction(cart.id, {
selected: [
{
id: "4",
value: 10904,
referenceValue: 10904,
planId: "c4ca4238a0b923820dcc509a6f75849b"
},
{
id: "1",
referenceValue: 60904,
value: 60904,
planId: "c4ca4238a0b923820dcc509a6f75849b"
}
]
})
}
Update Shipping Action
addresses: {
id?: string;
postalCode: string;
addressLine1?: string;
addressLine2?: string;
city?: string;
state?: string;
country: string;
}
id
: id of the address (for a new address the id is not required)
postalCode
: zip code of the address
addresLine1
: Represents the first line of the address, including the street number and name
addresLine2
: Provides additional address details, such as apartment, suite, or other relevant information for delivery
city
: City of the address
state
: State of the address
country
: Country of the address
export const ExampleComponent: CustomComponent = ({ cart, updateShippingAction}) => {
function updateShipping(){
updateShippingAction(cart.id, {
addresses: [
{
postalCode: "10018",
country: "USA",
addressLine1: "621 Garrett Island"
}
]
})
}
Update Delivery Action
selected: [{
id: string;
type: string;
addressId?: string;
items: string[];
}]
id
: ID of a delivery method
type
: Type of delivery method
addressId
: ID of the address selected for this delivery
items
: an array with lineIds
of the corresponding products that will be bundled in a package and shipped with this delivery method
Information of items
and delivery methods
can be retrieved from the cart
List of Addresses
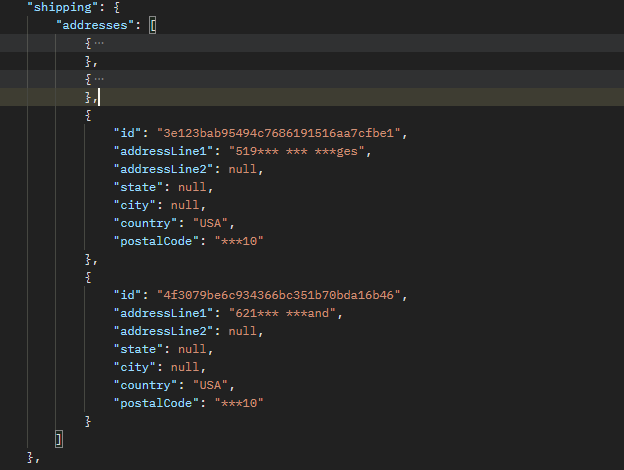
export const ExampleComponent: CustomComponent = ({ cart, updateDeliveryAction}) => {
function updateDelivery(){
updateDeliveryAction(cart.id, {
selected: [
{
id: "Express Delivery",
type: "delivery",
items: ["5cdd8e6391ae484a97251f474ded5659", "59625b8768fe4491bc7c216e09956209"],
addressId: "2"
},
{
id: "Standard Ground",
type: "delivery",
items: ["d9c857e52eb04ea083414d2845dd33af"],
addressId: "2"
}
]
})
}
Updated 7 months ago