How to create a custom Component
In this tutorial, we'll continue to explore the Medical Prescription customization. The first step will be to create the UI component.
For an overview of Components take a look at our Component section.
You'll notice that this component is completely separate from your commerce provider, and this is by design. Our goal with Ollie Shop is to allow merchants to build components without being tied to any foreign abstractions.
All Ollie Shop templates are composed of blocks. Think of these blocks as open slots where one can fit a component. For our example, we will be using a block that nested within the Product-Card. This way we don't have to re-write the entire Product-Card component.
As explained in the Component overview, an Ollie Shop component is composed of:
- an
index.tsx
Typescript file - a Tailwind
styles.css
file - the
package.json
file node_modules
folder (for external dependencies)
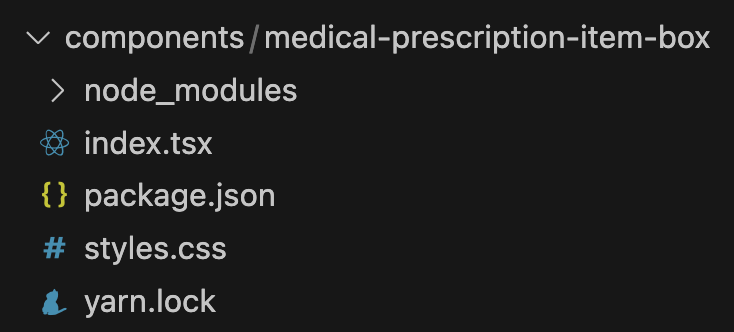
The package.json
file will include the basic information of your component
The styles.css
file is a simple declaration, if using Tailwind.

Below is how the index.tsx
file should look like. Notice how the React component has no context of the ecommerce provider. It is just exporting a CustomComponent.
import React from "react";
import "./styles.css";
import { CustomComponent } from "@ollieshop/sdk";
export const MedicalPrescriptionBox: CustomComponent = ({cart}) => {
return (
<>
{cart?.items.map((product: any) => (
<div className="ml-4 flex flex-1 flex-col justify-between sm:ml-6">
<>
<p className="mt-1 text-sm font-medium text-gray-900">
Please provide the URL for your prescription.
</p>
<span className="mt-4 flex space-x-2 text-sm text-gray-700" >
<input ref={ref} type="text" className="input-class-prescription" required style={{ padding: '10px 20px', border: '1px solid #007BFF', borderRadius: '5px' }} />
<button onClick={(event: React.FormEvent) } style={{ backgroundColor: '#007BFF', color: '#fff', padding: '10px 20px', border: 'none', borderRadius: '5px', cursor: 'pointer' }}>Send</button>
</span>
</>
</div>
)}
</>
);
};
export default MedicalPrescriptionBox;
However, the code above is nothing but a placeholder, given that when the user clicks "send" nothing happens.
We want the action of sending the data to already use the Ollie Shop API, in order to include the prescription data in the cart. So the next step will be to use the Ollie Shop Extensions API.
Updated about 1 year ago
Next you'll learn how to use the Extensions API to send data from your component to the Cart